FX自動売買ブログ pythonプログラミング勉強2回目
このブログは自動売買に関するプログラミングの知識を付けたい20代がノートのように投稿していくものです。※このカテゴリーのコードはChatGPTで作成されたものを元に、学習用として掲載しています。動作保証や商用利用はしていません。
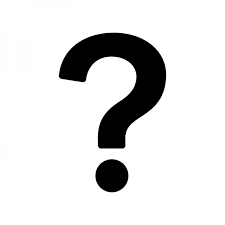
【この記事はこんな人が書いてます】デリバティブ取引は2021年8月から。週次最高120万円、月次最高ROI13,000%、月最高損40万のアマチュアトレーダー。仮想通貨/為替/ゴールドを取引。PythonとMQL4を勉強中。
前回のコードを用いて言葉と記号を覚えていきます
import MetaTrader4 as mt4
import time
import datetime
# ログイン情報(デモ口座の認証情報)
account = "your_account_number" # 例: "12345678"
password = "your_account_password"
server = "your_server_address" # 例: "demo.mt4broker.com"
# MT4に接続
def initialize_mt4():
if not mt4.initialize(account=account, password=password, server=server):
print("MT4 Initialization failed.")
return False
return True
# USDJPYを購入する関数
def buy_usd_jpy(lot_size=1):
symbol = "USDJPY"
price = mt4.symbol_info_tick(symbol).ask # 現在のask価格を取得
slippage = 10 # スリッページ設定
deviation = 20 # 最大許容変動
# 注文パラメータの設定
order = {
"action": mt4.TRADE_ACTION_DEAL,
"symbol": symbol,
"volume": lot_size,
"type": mt4.ORDER_TYPE_BUY,
"price": price,
"slippage": slippage,
"deviation": deviation,
"magic": 234000, # 任意のマジックナンバー
"comment": "Python Auto Buy",
"type_filling": mt4.ORDER_FILLING_IOC,
"type_time": mt4.ORDER_TIME_GTC
}
# 注文実行
result = mt4.order_send(order)
if result.retcode != mt4.TRADE_RETCODE_DONE:
print("Buy order failed:", result)
else:
print(f"Buy order executed: {result}")
# USDJPYを決済する関数
def sell_usd_jpy(lot_size=1):
symbol = "USDJPY"
price = mt4.symbol_info_tick(symbol).bid # 現在のbid価格を取得
slippage = 10
deviation = 20
# 決済注文パラメータの設定
order = {
"action": mt4.TRADE_ACTION_DEAL,
"symbol": symbol,
"volume": lot_size,
"type": mt4.ORDER_TYPE_SELL,
"price": price,
"slippage": slippage,
"deviation": deviation,
"magic": 234000,
"comment": "Python Auto Sell",
"type_filling": mt4.ORDER_FILLING_IOC,
"type_time": mt4.ORDER_TIME_GTC
}
# 注文実行
result = mt4.order_send(order)
if result.retcode != mt4.TRADE_RETCODE_DONE:
print("Sell order failed:", result)
else:
print(f"Sell order executed: {result}")
# 指定時間に取引を行うメイン関数
def trade_at_specified_time():
if not initialize_mt4():
return
while True:
current_time = datetime.datetime.now()
# 9時になったら購入
if current_time.hour == 9 and current_time.minute == 0:
print("Placing buy order at 9:00")
buy_usd_jpy(lot_size=1)
time.sleep(60) # 1分間待機(重複実行を防ぐ)
# 17時になったら決済
if current_time.hour == 17 and current_time.minute == 0:
print("Placing sell order at 17:00")
sell_usd_jpy(lot_size=1)
time.sleep(60) # 1分間待機(重複実行を防ぐ)
# 60秒待機
time.sleep(60)
# 実行
if __name__ == "__main__":
trade_at_specified_time()
スペースはコードを読みやすくするために使う。関数と()の間とか:の後はスペースを入れない。字下げをするときは4つのインデント(スペース)を空ける
〇buy_usd_jpy(lot_size=1)
☓buy_usd_jpy (lot_size=1)
def initialize_mt4():
if not mt4.initialize(account=account, password=password, server=server):
print("MT4 Initialization failed.")
return False
関数を定義
def
ifとelseで条件を定義
if temperature > 30:
print("It's hot!")
else:
print("It's not that hot.")
printで画面に出力
例 print("Hello, world!")
コメントを書くときに使う
#
変数に値を代入
=
条件文や関数の直後につける。ブロックの開始
:
値や引数を並べる
, 例 x,y=2,3
クラスやオブジェクトの呼び出し
.
複数のものを1つに格納するタプルや関数の引数を囲むときに使う
()
集合を定義
{} 例 order = {
"action": mt4.TRADE_ACTION_DEAL,
"symbol": symbol,
"volume": lot_size,
"type": mt4.ORDER_TYPE_BUY,
"price": price,
"slippage": slippage,
"deviation": deviation,
"magic": 234000, # 任意のマジックナンバー
"comment": "Python Auto Buy",
"type_filling": mt4.ORDER_FILLING_IOC,
"type_time": mt4.ORDER_TIME_GTC
}